A GtkComboBox is a widget that allows the user to choose from a list of valid choices.
The GtkComboBox displays the selected choice. When activated, the GtkComboBox displays a popup which allows the user to make a new
choice. The style in which the selected value is displayed, and the style of the popup is determined by the current theme. It may be
similar to a Windows-style combo box.
The GtkComboBox uses the model-view pattern; the list of valid choices is specified in the form of a tree model, and the display of the
choices can be adapted to the data in the model by using cell renderers, as you would in a tree view. This is possible since GtkComboBox
implements the CellLayout interface. The tree model holding the valid choices is not
restricted to a flat list, it can be a real tree, and the popup will reflect the tree structure.
To allow the user to enter values not in the model, the “has-entry” property allows the GtkComboBox to contain a
Entry. This entry can be accessed by calling
get_child on the combo box.
For a simple list of textual choices, the model-view API of GtkComboBox can be a bit overwhelming. In this case,
ComboBoxText offers a simple alternative. Both GtkComboBox and
ComboBoxText can contain an entry.
CSS nodes
combobox
├── box.linked
│ ╰── button.combo
│ ╰── box
│ ├── cellview
│ ╰── arrow
╰── window.popup
.linked class, a button with the .combo class and inside those buttons, there are a cellview and an arrow.
combobox
├── box.linked
│ ├── entry.combo
│ ╰── button.combo
│ ╰── box
│ ╰── arrow
╰── window.popup
SS node with name combobox. It contains a box with the .linked class. That box contains an entry and a button, both with the .combo class
added. The button also contains another node with name arrow.
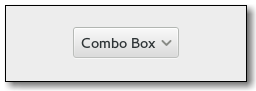
- public int active { get; set; }
The item which is currently active.
- public string? active_id { get; set; }
The value of the ID column of the active row.
- public bool add_tearoffs { get; set; }
The add-tearoffs property controls whether generated menus have
tearoff menu items.
- public SensitivityType button_sensitivity { get; set; }
Whether the dropdown button is sensitive when the model is empty.
- public CellArea cell_area { owned get; construct; }
The CellArea
used to layout cell renderers for this combo box.
- public int column_span_column { get; set; }
If this is set to a non-negative value, it must be the index of a
column of type g_type_int in the model.
- public int entry_text_column { get; set; }
The column in the combo box's model to associate with strings from the
entry if the combo was created with has_entry =
true.
- public bool has_entry { get; construct; }
Whether the combo box has an entry.
- public bool has_frame { get; set; }
The has-frame property controls whether a frame is drawn around the
entry.
- public int id_column { get; set; }
The column in the combo box's model that provides string IDs for the
values in the model, if != -1.
- public TreeModel model { get; set; }
The model from which the combo box takes the values shown in the list.
- public bool popup_fixed_width { get; set; }
Whether the popup's width should be a fixed width matching the
allocated width of the combo box.
- public bool popup_shown { get; }
Whether the combo boxes dropdown is popped up.
- public int row_span_column { get; set; }
If this is set to a non-negative value, it must be the index of a
column of type g_type_int in the model.
- public string tearoff_title { owned get; set; }
A title that may be displayed by the window manager when the popup is
torn-off.
- public int wrap_width { get; set; }
If wrap-width is set to a positive value, items in the popup will be
laid out along multiple columns, starting a new row on reaching the wrap width.
- public int get_active ()
Returns the index of the currently active item, or -1 if there’s no
active item.
- public unowned string? get_active_id ()
Returns the ID of the active row of this
.
- public bool get_active_iter (out TreeIter iter)
Sets iter
to point to the currently active item, if any
item is active.
- public bool get_add_tearoffs ()
- public SensitivityType get_button_sensitivity ()
Returns whether the combo box sets the dropdown button sensitive or
not when there are no items in the model.
- public int get_column_span_column ()
Returns the column with column span information for
this.
- public int get_entry_text_column ()
Returns the column which this is
using to get the strings from to display in the internal entry.
- public bool get_focus_on_click ()
Returns whether the combo box grabs focus when it is clicked with the
mouse.
- public bool get_has_entry ()
Returns whether the combo box has an entry.
- public int get_id_column ()
Returns the column which this is
using to get string IDs for values from.
- public unowned TreeModel get_model ()
Returns the TreeModel
which is acting as data source for this.
- public unowned Object get_popup_accessible ()
Gets the accessible object corresponding to the combo box’s popup.
- public bool get_popup_fixed_width ()
Gets whether the popup uses a fixed width matching the allocated width
of the combo box.
- public unowned TreeViewRowSeparatorFunc get_row_separator_func ()
Returns the current row separator function.
- public int get_row_span_column ()
Returns the column with row span information for
this.
- public unowned string get_title ()
Gets the current title of the menu in tearoff mode.
- public int get_wrap_width ()
Returns the wrap width which is used to determine the number of
columns for the popup menu.
- public void popup_for_device (Device device)
Pops up the menu or dropdown list of this
, the popup window will be grabbed so only device
and its associated pointer/keyboard are the only
Devices able to send events to it.
- public void set_active (int index_)
Sets the active item of this to be
the item at index
.
- public bool set_active_id (string? active_id)
Changes the active row of this to
the one that has an ID equal to active_id
, or unsets the active row if active_id
is
null.
- public void set_active_iter (TreeIter? iter)
Sets the current active item to be the one referenced by iter
, or unsets the active item if iter
is null.
- public void set_add_tearoffs (bool add_tearoffs)
Sets whether the popup menu should have a tearoff menu item.
- public void set_button_sensitivity (SensitivityType sensitivity)
Sets whether the dropdown button of the combo box should be always
sensitive (gtk_sensitivity_on), never sensitive (gtk_sensitivity_off
) or only if there is at least one item to display (gtk_sensitivity_auto).
- public void set_column_span_column (int column_span)
Sets the column with column span information for
this to be column_span
.
- public void set_entry_text_column (int text_column)
Sets the model column which this
should use to get strings from to be text_column
.
- public void set_focus_on_click (bool focus_on_click)
Sets whether the combo box will grab focus when it is clicked with the
mouse.
- public void set_id_column (int id_column)
Sets the model column which this
should use to get string IDs for values from.
- public void set_model (TreeModel? model)
Sets the model used by this to be
model
.
- public void set_popup_fixed_width (bool fixed)
Specifies whether the popup’s width should be a fixed width matching
the allocated width of the combo box.
- public void set_row_separator_func (owned TreeViewRowSeparatorFunc func)
Sets the row separator function, which is used to determine whether a
row should be drawn as a separator.
- public void set_row_span_column (int row_span)
Sets the column with row span information for
this to be row_span
.
- public void set_title (string title)
Sets the menu’s title in tearoff mode.
- public void set_wrap_width (int width)
Sets the wrap width of this to be
width
.