The Entry widget is a single line text entry widget.
A fairly large set of key bindings are supported by default. If the entered text is longer than the allocation of the widget, the widget
will scroll so that the cursor position is visible.
When using an entry for passwords and other sensitive information, it can be put into “password mode” using
set_visibility. In this mode, entered text is displayed using a “invisible”
character. By default, GTK+ picks the best invisible character that is available in the current font, but it can be changed with
set_invisible_char. Since 2.16, GTK+ displays a warning when Caps Lock or
input methods might interfere with entering text in a password entry. The warning can be turned off with the
caps_lock_warning property.
Since 2.16, GtkEntry has the ability to display progress or activity information behind the text. To make an entry display such
information, use set_progress_fraction or
set_progress_pulse_step.
Additionally, GtkEntry can show icons at either side of the entry. These icons can be activatable by clicking, can be set up as drag
source and can have tooltips. To add an icon, use set_icon_from_gicon or
one of the various other functions that set an icon from a stock id, an icon name or a pixbuf. To trigger an action when the user clicks
an icon, connect to the icon_press signal. To allow DND operations from an icon,
use set_icon_drag_source. To set a tooltip on an icon, use
set_icon_tooltip_text or the corresponding function for markup.
Note that functionality or information that is only available by clicking on an icon in an entry may not be accessible at all to users
which are not able to use a mouse or other pointing device. It is therefore recommended that any such functionality should also be
available by other means, e.g. via the context menu of the entry.
CSS nodes
entry[.read-only][.flat][.warning][.error]
├── image.left
├── image.right
├── undershoot.left
├── undershoot.right
├── [selection]
├── [progress[.pulse]]
╰── [window.popup]
ntry. Depending on the properties of the entry, the style classes .read-only and .flat may appear. The style classes .warning and .error
may also be used with entries.
When the entry shows icons, it adds subnodes with the name image and the style class .left or .right, depending on where the icon
appears.
When the entry has a selection, it adds a subnode with the name selection.
When the entry shows progress, it adds a subnode with the name progress. The node has the style class .pulse when the shown progress is
pulsing.
The CSS node for a context menu is added as a subnode below entry as well.
The undershoot nodes are used to draw the underflow indication when content is scrolled out of view. These nodes get the .left and .right
style classes added depending on where the indication is drawn.
When touch is used and touch selection handles are shown, they are using CSS nodes with name cursor-handle. They get the .top or .bottom
style class depending on where they are shown in relation to the selection. If there is just a single handle for the text cursor, it gets
the style class .insertion-cursor.
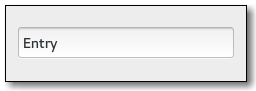
- public bool get_activates_default ()
- public float get_alignment ()
- public unowned AttrList? get_attributes ()
Gets the attribute list that was set on the entry using
set_attributes, if any.
- public unowned EntryBuffer get_buffer ()
Get the EntryBuffer
object which holds the text for this widget.
- public unowned EntryCompletion get_completion ()
Returns the auxiliary completion object currently in use by
this.
- public int get_current_icon_drag_source ()
Returns the index of the icon which is the source of the current DND
operation, or -1.
- public unowned Adjustment? get_cursor_hadjustment ()
Retrieves the horizontal cursor adjustment for the entry.
- public virtual void get_frame_size (out int x, out int y, out int width, out int height)
- public bool get_has_frame ()
- public bool get_icon_activatable (EntryIconPosition icon_pos)
Returns whether the icon is activatable.
- public Rectangle get_icon_area (EntryIconPosition icon_pos)
Gets the area where entry’s icon at icon_pos
is drawn.
- public int get_icon_at_pos (int x, int y)
Finds the icon at the given position and return its index.
- public unowned Icon? get_icon_gicon (EntryIconPosition icon_pos)
Retrieves the Icon used for the icon,
or null if there is no icon or if the icon was set by some other method (e.
- public unowned string? get_icon_name (EntryIconPosition icon_pos)
Retrieves the icon name used for the icon, or
null if there is no icon or if the icon was set by some other method (e.
- public unowned Pixbuf? get_icon_pixbuf (EntryIconPosition icon_pos)
Retrieves the image used for the icon.
- public bool get_icon_sensitive (EntryIconPosition icon_pos)
Returns whether the icon appears sensitive or insensitive.
- public unowned string get_icon_stock (EntryIconPosition icon_pos)
Retrieves the stock id used for the icon, or
null if there is no icon or if the icon was set by some other method (e.
- public ImageType get_icon_storage_type (EntryIconPosition icon_pos)
Gets the type of representation being used by the icon to store image
data.
- public string? get_icon_tooltip_markup (EntryIconPosition icon_pos)
Gets the contents of the tooltip on the icon at the specified position
in this.
- public string? get_icon_tooltip_text (EntryIconPosition icon_pos)
Gets the contents of the tooltip on the icon at the specified position
in this.
- public unowned Border? get_inner_border ()
- public InputHints get_input_hints ()
- public InputPurpose get_input_purpose ()
- public unichar get_invisible_char ()
Retrieves the character displayed in place of the real characters for
entries with visibility set to false.
- public unowned Layout get_layout ()
Gets the Layout used to display the entry.
- public void get_layout_offsets (out int x, out int y)
Obtains the position of the Layout used to
render text in the entry, in widget coordinates.
- public int get_max_length ()
Retrieves the maximum allowed length of the text in
this.
- public int get_max_width_chars ()
Retrieves the desired maximum width of this
, in characters.
- public bool get_overwrite_mode ()
- public unowned string get_placeholder_text ()
Retrieves the text that will be displayed when
this is empty and unfocused
- public double get_progress_fraction ()
Returns the current fraction of the task that’s been completed.
- public double get_progress_pulse_step ()
- public unowned TabArray? get_tabs ()
Gets the tabstops that were set on the entry using
set_tabs, if any.
- public unowned string get_text ()
Retrieves the contents of the entry widget.
- public void get_text_area (out Rectangle text_area)
Gets the area where the entry’s text is drawn.
- public virtual void get_text_area_size (out int x, out int y, out int width, out int height)
- public uint16 get_text_length ()
Retrieves the current length of the text in
this.
- public bool get_visibility ()
Retrieves whether the text in this
is visible.
- public int get_width_chars ()
- public void grab_focus_without_selecting ()
Causes this to have keyboard focus.
- public bool im_context_filter_keypress (EventKey event)
Allow the Entry input method to internally
handle key press and release events.
- public int layout_index_to_text_index (int layout_index)
Converts from a position in the entry’s Layout
(returned by get_layout) to a position in the entry contents (returned
by get_text).
- public void progress_pulse ()
Indicates that some progress is made, but you don’t know how much.
- public void reset_im_context ()
Reset the input method context of the entry if needed.
- public void set_activates_default (bool setting)
If setting
is true,
pressing Enter in the this will activate the default widget for the window containing the entry.
- public void set_alignment (float xalign)
Sets the alignment for the contents of the entry.
- public void set_attributes (AttrList attrs)
Sets a AttrList; the attributes in the list
are applied to the entry text.
- public void set_buffer (EntryBuffer buffer)
Set the EntryBuffer
object which holds the text for this widget.
- public void set_completion (EntryCompletion? completion)
Sets completion
to be the auxiliary completion object to
use with this.
- public void set_cursor_hadjustment (Adjustment? adjustment)
Hooks up an adjustment to the cursor position in an entry, so that
when the cursor is moved, the adjustment is scrolled to show that position.
- public void set_has_frame (bool setting)
Sets whether the entry has a beveled frame around it.
- public void set_icon_activatable (EntryIconPosition icon_pos, bool activatable)
Sets whether the icon is activatable.
- public void set_icon_drag_source (EntryIconPosition icon_pos, TargetList target_list, DragAction actions)
Sets up the icon at the given position so that GTK+ will start a drag
operation when the user clicks and drags the icon.
- public void set_icon_from_gicon (EntryIconPosition icon_pos, Icon? icon)
Sets the icon shown in the entry at the specified position from the
current icon theme.
- public void set_icon_from_icon_name (EntryIconPosition icon_pos, string? icon_name)
Sets the icon shown in the entry at the specified position from the
current icon theme.
- public void set_icon_from_pixbuf (EntryIconPosition icon_pos, Pixbuf? pixbuf)
Sets the icon shown in the specified position using a pixbuf.
- public void set_icon_from_stock (EntryIconPosition icon_pos, string? stock_id)
Sets the icon shown in the entry at the specified position from a
stock image.
- public void set_icon_sensitive (EntryIconPosition icon_pos, bool sensitive)
Sets the sensitivity for the specified icon.
- public void set_icon_tooltip_markup (EntryIconPosition icon_pos, string? tooltip)
Sets tooltip
as the contents of the tooltip for the icon
at the specified position.
- public void set_icon_tooltip_text (EntryIconPosition icon_pos, string? tooltip)
Sets tooltip
as the contents of the tooltip for the icon
at the specified position.
- public void set_inner_border (Border? border)
Sets entry’s inner-border property
to border
, or clears it if null is passed.
- public void set_input_hints (InputHints hints)
Sets the
input_hints property, which allows input methods to fine-tune their behaviour.
- public void set_input_purpose (InputPurpose purpose)
Sets the
input_purpose property which can be used by on-screen keyboards and other input methods to adjust their behaviour.
- public void set_invisible_char (unichar ch)
Sets the character to use in place of the actual text when
set_visibility has been called to set text visibility to
false.
- public void set_max_length (int max)
Sets the maximum allowed length of the contents of the widget.
- public void set_max_width_chars (int n_chars)
Sets the desired maximum width in characters of
this.
- public void set_overwrite_mode (bool overwrite)
Sets whether the text is overwritten when typing in the
Entry.
- public void set_placeholder_text (string? text)
Sets text to be displayed in this
when it is empty and unfocused.
- public void set_progress_fraction (double fraction)
Causes the entry’s progress indicator to “fill in” the given
fraction of the bar.
- public void set_progress_pulse_step (double fraction)
Sets the fraction of total entry width to move the progress bouncing
block for each call to progress_pulse.
- public void set_tabs (TabArray tabs)
Sets a TabArray; the tabstops in the array
are applied to the entry text.
- public void set_text (string text)
Sets the text in the widget to the given value, replacing the current
contents.
- public void set_visibility (bool visible)
Sets whether the contents of the entry are visible or not.
- public void set_width_chars (int n_chars)
Changes the size request of the entry to be about the right size for
n_chars
characters.
- public int text_index_to_layout_index (int text_index)
Converts from a position in the entry contents (returned by
get_text) to a position in the entry’s Layout (
returned by get_layout, with text retrieved via get_text
).
- public void unset_invisible_char ()
- public virtual signal void activate ()
The activate signal is emitted when the
user hits the Enter key.
- public virtual signal void backspace ()
The backspace signal is a keybinding
signal which gets emitted when the user asks for it.
- public virtual signal void copy_clipboard ()
The copy_clipboard signal is a
keybinding signal which gets emitted to copy the selection to the clipboard.
- public virtual signal void cut_clipboard ()
The cut_clipboard signal is a
keybinding signal which gets emitted to cut the selection to the clipboard.
- public virtual signal void delete_from_cursor (DeleteType type, int count)
The delete_from_cursor signal is a
keybinding signal which gets emitted when the user initiates a text deletion.
- public signal void icon_press (EntryIconPosition icon_pos, Event event)
The icon_press signal is emitted when an
activatable icon is clicked.
- public signal void icon_release (EntryIconPosition icon_pos, Event event)
The icon_release signal is emitted on the
button release from a mouse click over an activatable icon.
- public virtual signal void insert_at_cursor (string str)
The insert_at_cursor signal is a
keybinding signal which gets emitted when the user initiates the insertion of a fixed string at the cursor.
- public virtual signal void insert_emoji ()
The insert_emoji signal is a keybinding
signal which gets emitted to present the Emoji chooser for the entry
.
- public virtual signal void move_cursor (MovementStep step, int count, bool extend_selection)
The move_cursor signal is a keybinding
signal which gets emitted when the user initiates a cursor movement.
- public virtual signal void paste_clipboard ()
The paste_clipboard signal is a
keybinding signal which gets emitted to paste the contents of the clipboard into the text view.
- public virtual signal void populate_popup (Menu popup)
The populate_popup signal gets emitted
before showing the context menu of the entry.
- public signal void preedit_changed (string preedit)
If an input method is used, the typed text will not immediately be
committed to the buffer.
- public virtual signal void toggle_overwrite ()
The toggle_overwrite signal is a
keybinding signal which gets emitted to toggle the overwrite mode of the entry.