The GtkAboutDialog offers a simple way to display information about a program like its logo, name, copyright, website and license.
It is also possible to give credits to the authors, documenters, translators and artists who have worked on the program. An about dialog
is typically opened when the user selects the `About` option from the `Help` menu. All parts of the dialog are optional.
About dialogs often contain links and email addresses. GtkAboutDialog displays these as clickable links. By default, it calls
show_uri_on_window when a user clicks one. The behaviour can be overridden with
the activate_link signal.
To specify a person with an email address, use a string like "Edgar Allan Poe <[email protected]>". To specify a website with a title,
use a string like "GTK+ team http://www.gtk.org".
To make constructing a GtkAboutDialog as convenient as possible, you can use the function
show_about_dialog which constructs and shows a dialog and keeps it around so that
it can be shown again.
Note that GTK+ sets a default title of `_("About s")` on the dialog window (where %s is replaced by the
name of the application, but in order to ensure proper translation of the title, applications should set the title property explicitly
when constructing a GtkAboutDialog, as shown in the following example:
GdkPixbuf *example_logo = gdk_pixbuf_new_from_file ("./logo.png", NULL);
gtk_show_about_dialog (NULL,
"program-name", "ExampleCode",
"logo", example_logo,
"title", _("About ExampleCode"),
NULL);
It is also possible to show a AboutDialog like any other Dialog,
e.g. using run. In this case, you might need to know that the “Close” button returns
the CANCEL response id.
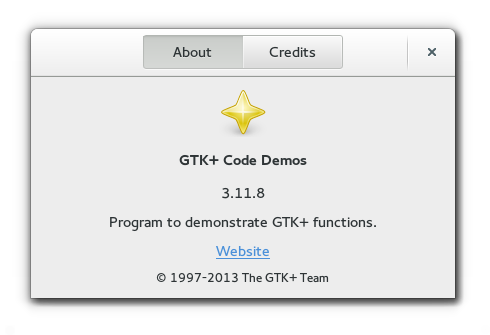
Example: AboutDialog:
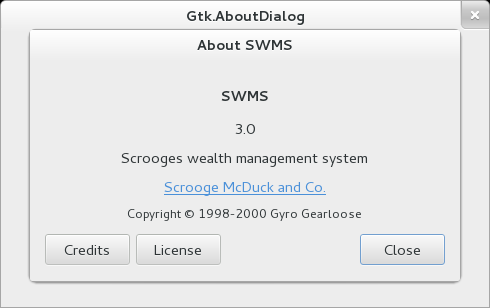
public static int main (string[] args) {
Gtk.init (ref args);
// Create a window:
Gtk.Window window = new Gtk.Window ();
window.destroy.connect (Gtk.main_quit);
window.set_default_size (500, 500);
window.show_all ();
// Configure the dialog:
Gtk.AboutDialog dialog = new Gtk.AboutDialog ();
dialog.set_destroy_with_parent (true);
dialog.set_transient_for (window);
dialog.set_modal (true);
dialog.artists = {"Darkwing Duck", "Launchpad McQuack"};
dialog.authors = {"Scrooge McDuck", "Gyro Gearloose"};
dialog.documenters = null; // Real inventors don't document.
dialog.translator_credits = null; // We only need a scottish version.
dialog.program_name = "SWMS";
dialog.comments = "Scrooges wealth management system";
dialog.copyright = "Copyright © 1998-2000 Gyro Gearloose";
dialog.version = "3.0";
dialog.license = "Permission is hereby granted, NOT free of charge, ..., very long text";
dialog.wrap_license = true;
dialog.website = "http://en.wikipedia.org/wiki/Scrooge_McDuck";
dialog.website_label = "Scrooge McDuck and Co.";
dialog.response.connect ((response_id) => {
if (response_id == Gtk.ResponseType.CANCEL || response_id == Gtk.ResponseType.DELETE_EVENT) {
dialog.hide_on_delete ();
}
});
// Show the dialog:
dialog.present ();
Gtk.main ();
return 0;
}
valac --pkg gtk+-3.0 Gtk.AboutDialog.vala
- public string[] artists { get; set; }
The people who contributed artwork to the program, as a
null-terminated array of strings.
- public string[] authors { get; set; }
The authors of the program, as a null
-terminated array of strings.
- public string comments { get; set; }
Comments about the program.
- public string copyright { get; set; }
Copyright information for the program.
- public string[] documenters { get; set; }
The people documenting the program, as a
null-terminated array of strings.
- public string license { get; set; }
The license of the program.
- public License license_type { get; set; }
The license of the program, as a value of the
gtklicense enumeration.
- public Pixbuf logo { get; set; }
A logo for the about box.
- public string logo_icon_name { get; set; }
A named icon to use as the logo for the about box.
- public string program_name { get; set; }
The name of the program.
- public string translator_credits { get; set; }
Credits to the translators.
- public string version { get; set; }
The version of the program.
- public string website { get; set; }
The URL for the link to the website of the program.
- public string website_label { get; set; }
The label for the link to the website of the program.
- public bool wrap_license { get; set; }
Whether to wrap the text in the license dialog.
- public void add_credit_section (string section_name, string[] people)
Creates a new section in the Credits page.
- public unowned string[] get_artists ()
Returns the string which are displayed in the artists tab of the
secondary credits dialog.
- public unowned string[] get_authors ()
Returns the string which are displayed in the authors tab of the
secondary credits dialog.
- public unowned string get_comments ()
Returns the comments string.
- public unowned string get_copyright ()
Returns the copyright string.
- public unowned string[] get_documenters ()
Returns the string which are displayed in the documenters tab of the
secondary credits dialog.
- public unowned string get_license ()
Returns the license information.
- public License get_license_type ()
- public unowned Pixbuf get_logo ()
Returns the pixbuf displayed as logo in the about dialog.
- public unowned string get_logo_icon_name ()
Returns the icon name displayed as logo in the about dialog.
- public unowned string get_program_name ()
Returns the program name displayed in the about dialog.
- public unowned string get_translator_credits ()
Returns the translator credits string which is displayed in the
translators tab of the secondary credits dialog.
- public unowned string get_version ()
Returns the version string.
- public unowned string get_website ()
Returns the website URL.
- public unowned string get_website_label ()
Returns the label used for the website link.
- public bool get_wrap_license ()
Returns whether the license text in this
is automatically wrapped.
- public void set_artists (string[] artists)
Sets the strings which are displayed in the artists tab of the
secondary credits dialog.
- public void set_authors (string[] authors)
Sets the strings which are displayed in the authors tab of the
secondary credits dialog.
- public void set_comments (string? comments)
Sets the comments string to display in the about dialog.
- public void set_copyright (string? copyright)
Sets the copyright string to display in the about dialog.
- public void set_documenters (string[] documenters)
Sets the strings which are displayed in the documenters tab of the
secondary credits dialog.
- public void set_license (string? license)
Sets the license information to be displayed in the secondary license
dialog.
- public void set_license_type (License license_type)
Sets the license of the application showing the
this dialog from a list of known licenses.
- public void set_logo (Pixbuf? logo)
Sets the pixbuf to be displayed as logo in the about dialog.
- public void set_logo_icon_name (string? icon_name)
Sets the pixbuf to be displayed as logo in the about dialog.
- public void set_program_name (string name)
Sets the name to display in the about dialog.
- public void set_translator_credits (string? translator_credits)
Sets the translator credits string which is displayed in the
translators tab of the secondary credits dialog.
- public void set_version (string? version)
Sets the version string to display in the about dialog.
- public void set_website (string? website)
Sets the URL to use for the website link.
- public void set_website_label (string website_label)
Sets the label to be used for the website link.
- public void set_wrap_license (bool wrap_license)
Sets whether the license text in this
is automatically wrapped.