A GtkWindow is a toplevel window which can contain other widgets.
Windows normally have decorations that are under the control of the windowing system and allow the user to manipulate the window (resize
it, move it, close it,...).
GtkWindow as GtkBuildable
The GtkWindow implementation of the Buildable interface supports a custom `<
accel-groups>` element, which supports any number of `<group>` elements representing the
AccelGroup objects you want to add to your window (synonymous with
add_accel_group.
It also supports the `<initial-focus>` element, whose name property names the widget to receive the focus when the window is
mapped.
An example of a UI definition fragment with accel groups:
<object class="GtkWindow">
<accel-groups>
<group name="accelgroup1"/>
</accel-groups>
<initial-focus name="thunderclap"/>
</object>
...
<object class="GtkAccelGroup" id="accelgroup1"/>
The GtkWindow implementation of the Buildable interface supports setting a child as
the titlebar by specifying “titlebar” as the “type” attribute of a `<child>` element.
CSS nodes
window.background
├── decoration
├── <titlebar child>.titlebar [.default-decoration]
╰── <child>
main CSS node with name window and style class .background, and a subnode with name decoration.
Style classes that are typically used with the main CSS node are .csd (when client-side decorations are in use), .solid-csd (for
client-side decorations without invisible borders), .ssd (used by mutter when rendering server-side decorations). GtkWindow also
represents window states with the following style classes on the main node: .tiled, .maximized, .fullscreen. Specialized types of window
often add their own discriminating style classes, such as .popup or .tooltip.
GtkWindow adds the .titlebar and .default-decoration style classes to the widget that is added as a titlebar child.
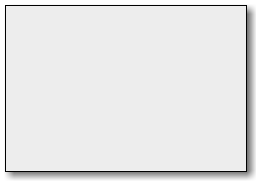
- public bool activate_key (EventKey event)
Activates mnemonics and accelerators for this
Window.
- public void add_accel_group (AccelGroup accel_group)
Associate accel_group
with
this, such that calling accel_groups_activate on
this will activate accelerators in accel_group
.
- public void add_mnemonic (uint keyval, Widget target)
Adds a mnemonic to this window.
- public void begin_move_drag (int button, int root_x, int root_y, uint32 timestamp)
Starts moving a window.
- public void begin_resize_drag (WindowEdge edge, int button, int root_x, int root_y, uint32 timestamp)
Starts resizing a window.
- public void close ()
Requests that the window is closed, similar to what happens when a
window manager close button is clicked.
- public void deiconify ()
Asks to deiconify (i.
- public void fullscreen ()
Asks to place this in the fullscreen
state.
- public void fullscreen_on_monitor (Screen screen, int monitor)
Asks to place this in the fullscreen
state.
- public bool get_accept_focus ()
- public unowned Application? get_application ()
Gets the Application
associated with the window (if any).
- public unowned Widget? get_attached_to ()
Fetches the attach widget for this window.
- public bool get_decorated ()
Returns whether the window has been set to have decorations such as a
title bar via set_decorated.
- public void get_default_size (out int width, out int height)
Gets the default size of the window.
- public unowned Widget? get_default_widget ()
Returns the default widget for this.
- public bool get_deletable ()
Returns whether the window has been set to have a close button via
set_deletable.
- public bool get_destroy_with_parent ()
Returns whether the window will be destroyed with its transient
parent.
- public unowned Widget? get_focus ()
Retrieves the current focused widget within the window.
- public bool get_focus_on_map ()
- public bool get_focus_visible ()
- public Gravity get_gravity ()
- public unowned WindowGroup get_group ()
Returns the group for this or the
default group, if this is null or if
this does not have an explicit window group.
- public bool get_has_resize_grip ()
Determines whether the window may have a resize grip.
- public bool get_hide_titlebar_when_maximized ()
Returns whether the window has requested to have its titlebar hidden
when maximized.
- public unowned Pixbuf? get_icon ()
Gets the value set by
set_icon (or if you've called
set_icon_list, gets the first icon in the icon list).
- public List<unowned Pixbuf> get_icon_list ()
- public unowned string? get_icon_name ()
Returns the name of the themed icon for the window, see
set_icon_name.
- public ModifierType get_mnemonic_modifier ()
Returns the mnemonic modifier for this window.
- public bool get_mnemonics_visible ()
- public bool get_modal ()
Returns whether the window is modal.
- public double get_opacity ()
Fetches the requested opacity for this window.
- public void get_position (out int root_x, out int root_y)
This function returns the position you need to pass to
move to keep this in its current position.
- public bool get_resizable ()
- public bool get_resize_grip_area (out Rectangle rect)
If a window has a resize grip, this will retrieve the grip position,
width and height into the specified Rectangle.
- public unowned string? get_role ()
Returns the role of the window.
- public unowned Screen get_screen ()
Returns the Screen associated with
this.
- public void get_size (out int width, out int height)
Obtains the current size of this.
- public bool get_skip_pager_hint ()
- public bool get_skip_taskbar_hint ()
- public unowned string? get_title ()
Retrieves the title of the window.
- public unowned Widget? get_titlebar ()
Returns the custom titlebar that has been set with
set_titlebar.
- public unowned Window? get_transient_for ()
Fetches the transient parent for this window.
- public WindowTypeHint get_type_hint ()
Gets the type hint for this window.
- public bool get_urgency_hint ()
- public WindowType get_window_type ()
Gets the type of the window.
- public bool has_group ()
Returns whether this has an explicit
window group.
- public void iconify ()
Asks to iconify (i.
- public void maximize ()
Asks to maximize this, so that it
becomes full-screen.
- public bool mnemonic_activate (uint keyval, ModifierType modifier)
Activates the targets associated with the mnemonic.
- public void move (int x, int y)
Asks the window manager to move this
to the given position.
- public bool parse_geometry (string geometry)
Parses a standard X Window System geometry string - see the manual
page for X (type “man X”) for details on this.
- public void present ()
Presents a window to the user.
- public void present_with_time (uint32 timestamp)
Presents a window to the user.
- public bool propagate_key_event (EventKey event)
Propagate a key press or release event to the focus widget and up the
focus container chain until a widget handles event
.
- public void remove_accel_group (AccelGroup accel_group)
- public void remove_mnemonic (uint keyval, Widget target)
Removes a mnemonic from this window.
- public void reshow_with_initial_size ()
Hides this, then reshows it,
resetting the default size and position of the window.
- public void resize (int width, int height)
Resizes the window as if the user had done so, obeying geometry
constraints.
- public bool resize_grip_is_visible ()
Determines whether a resize grip is visible for the specified window.
- public void resize_to_geometry (int width, int height)
Like resize, but
width
and height
are interpreted in terms of the base size and increment set with
gtk_window_set_geometry_hints.
- public void set_accept_focus (bool setting)
Windows may set a hint asking the desktop environment not to receive
the input focus.
- public void set_application (Application? application)
Sets or unsets the
Application associated with the window.
- public void set_attached_to (Widget? attach_widget)
Marks this as attached to
attach_widget
.
- public void set_decorated (bool setting)
By default, windows are decorated with a title bar, resize controls,
etc.
- public void set_default (Widget? default_widget)
The default widget is the widget that’s activated when the user
presses Enter in a dialog (for example).
- public void set_default_geometry (int width, int height)
Like
set_default_size, but width
and height
are interpreted in terms of the base size and increment set
with gtk_window_set_geometry_hints.
- public void set_default_size (int width, int height)
Sets the default size of a window.
- public void set_deletable (bool setting)
By default, windows have a close button in the window frame.
- public void set_destroy_with_parent (bool setting)
If setting
is true,
then destroying the transient parent of this will also destroy this
itself.
- public void set_focus_on_map (bool setting)
Windows may set a hint asking the desktop environment not to receive
the input focus when the window is mapped.
- public void set_focus_visible (bool setting)
- public void set_geometry_hints (Widget? geometry_widget, Geometry? geometry, WindowHints geom_mask)
This function sets up hints about how a window can be resized by the
user.
- public void set_gravity (Gravity gravity)
Window gravity defines the meaning of coordinates passed to
move.
- public void set_has_resize_grip (bool value)
Sets whether this has a corner
resize grip.
- public void set_has_user_ref_count (bool setting)
Tells GTK+ whether to drop its extra reference to the window when
destroy is called.
- public void set_hide_titlebar_when_maximized (bool setting)
If setting
is true,
then this will request that it’s titlebar should be hidden when maximized.
- public void set_icon (Pixbuf? icon)
Sets up the icon representing a Window.
- public bool set_icon_from_file (string filename) throws Error
Sets the icon for this.
- public void set_icon_list (List<Pixbuf> list)
Sets up the icon representing a Window.
- public void set_icon_name (string? name)
Sets the icon for the window from a named themed icon.
- public void set_keep_above (bool setting)
Asks to keep this above, so that it
stays on top.
- public void set_keep_below (bool setting)
Asks to keep this below, so that it
stays in bottom.
- public void set_mnemonic_modifier (ModifierType modifier)
Sets the mnemonic modifier for this window.
- public void set_mnemonics_visible (bool setting)
- public void set_modal (bool modal)
Sets a window modal or non-modal.
- public void set_opacity (double opacity)
Request the windowing system to make this
partially transparent, with opacity 0 being fully transparent and 1 fully opaque.
- public void set_position (WindowPosition position)
Sets a position constraint for this window.
- public void set_resizable (bool resizable)
Sets whether the user can resize a window.
- public void set_role (string role)
This function is only useful on X11, not with other GTK+ targets.
- public void set_screen (Screen screen)
Sets the Screen where the
this is displayed; if the window is already mapped, it will be unmapped, and then remapped on the
new screen.
- public void set_skip_pager_hint (bool setting)
Windows may set a hint asking the desktop environment not to display
the window in the pager.
- public void set_skip_taskbar_hint (bool setting)
Windows may set a hint asking the desktop environment not to display
the window in the task bar.
- public void set_startup_id (string startup_id)
Startup notification identifiers are used by desktop environment to
track application startup, to provide user feedback and other features.
- public void set_title (string title)
Sets the title of the Window.
- public void set_titlebar (Widget? titlebar)
Sets a custom titlebar for this.
- public void set_transient_for (Window? parent)
Dialog windows should be set transient for the main application window
they were spawned from.
- public void set_type_hint (WindowTypeHint hint)
By setting the type hint for the window, you allow the window manager
to decorate and handle the window in a way which is suitable to the function of the window in your application.
- public void set_urgency_hint (bool setting)
Windows may set a hint asking the desktop environment to draw the
users attention to the window.
- public void set_wmclass (string wmclass_name, string wmclass_class)
Don’t use this function.
- public void stick ()
Asks to stick this, which means that
it will appear on all user desktops.
- public bool try_activate_default ()
- public bool try_activate_focus ()
- public void unfullscreen ()
Asks to toggle off the fullscreen state for
this.
- public void unmaximize ()
Asks to unmaximize this.
- public void unstick ()
Asks to unstick this, which means
that it will appear on only one of the user’s desktops.