GtkScrolledWindow is a container that accepts a single child widget, makes that child scrollable using either internally added scrollbars
or externally associated adjustments, and optionally draws a frame around the child.
Widgets with native scrolling support, i.e. those whose classes implement the Scrollable
interface, are added directly. For other types of widget, the class Viewport acts as an
adaptor, giving scrollability to other widgets. GtkScrolledWindow’s implementation of
add intelligently accounts for whether or not the added child is a Scrollable.
If it isn’t, ScrolledWindow wraps the child in a Viewport and
adds that for you. Therefore, you can just add any child widget and not worry about the details.
If add has added a Viewport for you,
you can remove both your added child widget from the Viewport, and the
Viewport from the GtkScrolledWindow, like this:
GtkWidget *scrolled_window = gtk_scrolled_window_new (NULL, NULL);
GtkWidget *child_widget = gtk_button_new ();
// GtkButton is not a GtkScrollable, so GtkScrolledWindow will automatically
// add a GtkViewport.
gtk_container_add (GTK_CONTAINER (scrolled_window),
child_widget);
// Either of these will result in child_widget being unparented:
gtk_container_remove (GTK_CONTAINER (scrolled_window),
child_widget);
// or
gtk_container_remove (GTK_CONTAINER (scrolled_window),
gtk_bin_get_child (GTK_BIN (scrolled_window)));
Unless GtkScrolledWindow:policy
is GTK_POLICY_NEVER or GTK_POLICY_EXTERNAL, GtkScrolledWindow adds internal
Scrollbar widgets around its child. The scroll position of the child, and if applicable the
scrollbars, is controlled by the hadjustment and
vadjustment that are associated with the GtkScrolledWindow. See the
docs on Scrollbar for the details, but note that the “step_increment” and
“page_increment” fields are only effective if the policy causes scrollbars to be present.
If a GtkScrolledWindow doesn’t behave quite as you would like, or doesn’t have exactly the right layout, it’s very possible to set
up your own scrolling with Scrollbar and for example a
Grid.
Touch support
GtkScrolledWindow has built-in support for touch devices. When a touchscreen is used, swiping will move the scrolled window, and will
expose 'kinetic' behavior. This can be turned off with the
kinetic_scrolling property if it is undesired.
GtkScrolledWindow also displays visual 'overshoot' indication when the content is pulled beyond the end, and this situation can be
captured with the edge_overshot signal.
If no mouse device is present, the scrollbars will overlayed as narrow, auto-hiding indicators over the content. If traditional
scrollbars are desired although no mouse is present, this behaviour can be turned off with the
overlay_scrolling property.
CSS nodes
GtkScrolledWindow has a main CSS node with name scrolledwindow.
It uses subnodes with names overshoot and undershoot to draw the overflow and underflow indications. These nodes get the .left, .right,
.top or .bottom style class added depending on where the indication is drawn.
GtkScrolledWindow also sets the positional style classes (.left, .right, .top, .bottom) and style classes related to overlay scrolling (
.overlay-indicator, .dragging, .hovering) on its scrollbars.
If both scrollbars are visible, the area where they meet is drawn with a subnode named junction.
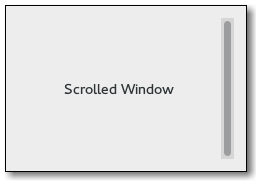
- public void add_with_viewport (Widget child)
Used to add children without native scrolling capabilities.
- public bool get_capture_button_press ()
Return whether button presses are captured during kinetic scrolling.
- public unowned Adjustment get_hadjustment ()
Returns the horizontal scrollbar’s adjustment, used to connect the
horizontal scrollbar to the child widget’s horizontal scroll functionality.
- public unowned Widget get_hscrollbar ()
Returns the horizontal scrollbar of this
.
- public bool get_kinetic_scrolling ()
Returns the specified kinetic scrolling behavior.
- public int get_max_content_height ()
Returns the maximum content height set.
- public int get_max_content_width ()
Returns the maximum content width set.
- public int get_min_content_height ()
Gets the minimal content height of this
, or -1 if not set.
- public int get_min_content_width ()
Gets the minimum content width of this
, or -1 if not set.
- public bool get_overlay_scrolling ()
Returns whether overlay scrolling is enabled for this scrolled window.
- public CornerType get_placement ()
Gets the placement of the contents with respect to the scrollbars for
the scrolled window.
- public void get_policy (out PolicyType hscrollbar_policy, out PolicyType vscrollbar_policy)
Retrieves the current policy values for the horizontal and vertical
scrollbars.
- public bool get_propagate_natural_height ()
Reports whether the natural height of the child will be calculated and
propagated through the scrolled window’s requested natural height.
- public bool get_propagate_natural_width ()
Reports whether the natural width of the child will be calculated and
propagated through the scrolled window’s requested natural width.
- public ShadowType get_shadow_type ()
Gets the shadow type of the scrolled window.
- public unowned Adjustment get_vadjustment ()
Returns the vertical scrollbar’s adjustment, used to connect the
vertical scrollbar to the child widget’s vertical scroll functionality.
- public unowned Widget get_vscrollbar ()
Returns the vertical scrollbar of this
.
- public void set_capture_button_press (bool capture_button_press)
Changes the behaviour of this with
regard to the initial event that possibly starts kinetic scrolling.
- public void set_hadjustment (Adjustment? hadjustment)
- public void set_kinetic_scrolling (bool kinetic_scrolling)
Turns kinetic scrolling on or off.
- public void set_max_content_height (int height)
Sets the maximum height that this
should keep visible.
- public void set_max_content_width (int width)
Sets the maximum width that this
should keep visible.
- public void set_min_content_height (int height)
Sets the minimum height that this
should keep visible.
- public void set_min_content_width (int width)
Sets the minimum width that this
should keep visible.
- public void set_overlay_scrolling (bool overlay_scrolling)
Enables or disables overlay scrolling for this scrolled window.
- public void set_placement (CornerType window_placement)
Sets the placement of the contents with respect to the scrollbars for
the scrolled window.
- public void set_policy (PolicyType hscrollbar_policy, PolicyType vscrollbar_policy)
Sets the scrollbar policy for the horizontal and vertical scrollbars.
- public void set_propagate_natural_height (bool propagate)
Sets whether the natural height of the child should be calculated and
propagated through the scrolled window’s requested natural height.
- public void set_propagate_natural_width (bool propagate)
Sets whether the natural width of the child should be calculated and
propagated through the scrolled window’s requested natural width.
- public void set_shadow_type (ShadowType type)
Changes the type of shadow drawn around the contents of
this.
- public void set_vadjustment (Adjustment? vadjustment)
- public void unset_placement ()
Unsets the placement of the contents with respect to the scrollbars
for the scrolled window.