GLArea is a widget that allows drawing with OpenGL.
GLArea sets up its own GLContext for the window it creates, and creates a
custom GL framebuffer that the widget will do GL rendering onto. It also ensures that this framebuffer is the default GL rendering target
when rendering.
In order to draw, you have to connect to the render signal, or subclass
GLArea and override the GtkGLAreaClass
.render() virtual function.
The GLArea widget ensures that the GLContext is associated with the
widget's drawing area, and it is kept updated when the size and position of the drawing area changes.
Drawing with GtkGLArea
The simplest way to draw using OpenGL commands in a GLArea is to create a widget instance and connect to the
render signal:
// create a GtkGLArea instance
GtkWidget *gl_area = gtk_gl_area_new ();
// connect to the "render" signal
g_signal_connect (gl_area, "render", G_CALLBACK (render), NULL);
The `render()` function will be called when the GLArea is ready for you to draw its content:
static gboolean
render (GtkGLArea *area, GdkGLContext *context)
{
// inside this function it's safe to use GL; the given
// #GdkGLContext has been made current to the drawable
// surface used by the #GtkGLArea and the viewport has
// already been set to be the size of the allocation
// we can start by clearing the buffer
glClearColor (0, 0, 0, 0);
glClear (GL_COLOR_BUFFER_BIT);
// draw your object
draw_an_object ();
// we completed our drawing; the draw commands will be
// flushed at the end of the signal emission chain, and
// the buffers will be drawn on the window
return TRUE;
}
If you need to initialize OpenGL state, e.g. buffer objects or shaders, you should use the
realize signal; you can use the
unrealize signal to clean up. Since the GLContext creation and initialization may fail, you will
need to check for errors, using get_error. An example of how to safely initialize
the GL state is:
static void
on_realize (GtkGLarea *area)
{
// We need to make the context current if we want to
// call GL API
gtk_gl_area_make_current (area);
// If there were errors during the initialization or
// when trying to make the context current, this
// function will return a #GError for you to catch
if (gtk_gl_area_get_error (area) != NULL)
return;
// You can also use gtk_gl_area_set_error() in order
// to show eventual initialization errors on the
// GtkGLArea widget itself
GError *internal_error = NULL;
init_buffer_objects (&error);
if (error != NULL)
{
gtk_gl_area_set_error (area, error);
g_error_free (error);
return;
}
init_shaders (&error);
if (error != NULL)
{
gtk_gl_area_set_error (area, error);
g_error_free (error);
return;
}
}
If you need to change the options for creating the GLContext you should use the
create_context signal.
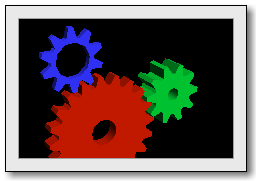
- public void attach_buffers ()
Ensures that the this framebuffer
object is made the current draw and read target, and that all the required buffers for the this are
created and bound to the frambuffer.
- public bool get_auto_render ()
Returns whether the area is in auto render mode or not.
- public unowned GLContext get_context ()
Retrieves the GLContext used by
this.
- public unowned Error? get_error ()
Gets the current error set on the this
.
- public bool get_has_alpha ()
Returns whether the area has an alpha component.
- public bool get_has_depth_buffer ()
Returns whether the area has a depth buffer.
- public bool get_has_stencil_buffer ()
Returns whether the area has a stencil buffer.
- public void get_required_version (out int major, out int minor)
- public bool get_use_es ()
- public void make_current ()
Ensures that the GLContext used by
this is associated with the GLArea.
- public void queue_render ()
Marks the currently rendered data (if any) as invalid, and queues a
redraw of the widget, ensuring that the render signal is emitted during the draw.
- public void set_auto_render (bool auto_render)
If auto_render
is true
the render signal will be emitted every time the widget draws.
- public void set_error (Error? error)
Sets an error on the area which will be shown instead of the GL
rendering.
- public void set_has_alpha (bool has_alpha)
If has_alpha
is true
the buffer allocated by the widget will have an alpha channel component, and when rendering to the window the result will be
composited over whatever is below the widget.
- public void set_has_depth_buffer (bool has_depth_buffer)
If has_depth_buffer
is true
the widget will allocate and enable a depth buffer for the target framebuffer.
- public void set_has_stencil_buffer (bool has_stencil_buffer)
If has_stencil_buffer
is true
the widget will allocate and enable a stencil buffer for the target framebuffer.
- public void set_required_version (int major, int minor)
Sets the required version of OpenGL to be used when creating the
context for the widget.
- public void set_use_es (bool use_es)
Sets whether the this should create
an OpenGL or an OpenGL ES context.