Notification
Object Hierarchy:
Description:
[ CCode ( type_id = "notify_notification_get_type ()" ) ]
public class Notification : Object
A passive pop-up notification.
Example: A basic notification:

public static int main (string[] args) {
string summary = "Short summary";
string body = "A long description";
// = Gtk.Stock.DIALOG_INFO
string icon = "dialog-information";
Notify.init ("My test app");
try {
Notify.Notification notification = new Notify.Notification (summary, body, icon);
notification.show ();
} catch (Error e) {
error ("Error: %s", e.message);
}
return 0;
}
valac --pkg libnotify basic.vala
Example: Notification with a button:
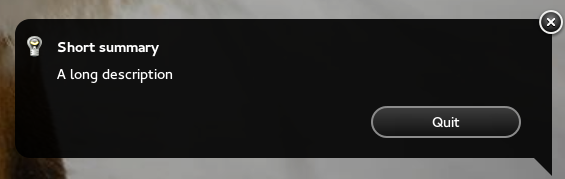
public static int main (string[] args) {
string summary = "Short summary";
string body = "A long description";
// = Gtk.Stock.DIALOG_INFO
string icon = "dialog-information";
Notify.init ("My test app");
GLib.MainLoop loop = new GLib.MainLoop ();
try {
Notify.Notification notification = new Notify.Notification (summary, body, icon);
notification.add_action ("action-name", "Quit", (notification, action) => {
print ("Bye!\n");
try {
notification.close ();
} catch (Error e) {
debug ("Error: %s", e.message);
}
loop.quit ();
});
notification.show ();
loop.run ();
} catch (Error e) {
error ("Error: %s", e.message);
}
return 0;
}
valac --pkg libnotify with-button.vala
Content:
Properties:
- public string app_name { owned get; set; }
- public string body { owned get; set construct; }
- public int closed_reason { get; }
- public string icon_name { owned get; set construct; }
- public int id { get; set construct; }
- public string summary { owned get; set construct; }
Creation methods:
Methods:
- public void add_action (string action, string label, owned ActionCallback callback)
Adds an action to a notification.
- public void clear_actions ()
Clears all actions from the notification.
- public void clear_hints ()
Clears all hints from the notification.
- public bool close () throws Error
Synchronously tells the notification server to hide the notification
on the screen.
- public unowned string get_activation_token ()
If an an action is currently being activated, return the activation
token.
- public int get_closed_reason ()
Returns the closed reason code for the notification.
- public void set_app_name (string app_name)
Sets the application name for the notification.
- public void set_category (string category)
Sets the category of this notification.
- public void set_hint (string key, Variant? value)
Sets a hint for key
with value value
.
- public void set_hint_byte (string key, uchar value)
Sets a hint with a byte value.
- public void set_hint_byte_array (string key, uchar[] value)
Sets a hint with a byte array value.
- public void set_hint_double (string key, double value)
Sets a hint with a double value.
- public void set_hint_int32 (string key, int value)
Sets a hint with a 32-bit integer value.
- public void set_hint_string (string key, string value)
Sets a hint with a string value.
- public void set_hint_uint32 (string key, uint value)
Sets a hint with an unsigned 32-bit integer value.
- public void set_icon_from_pixbuf (Pixbuf icon)
Sets the icon in the notification from a Pixbuf
.
- public void set_image_from_pixbuf (Pixbuf pixbuf)
Sets the image in the notification from a Pixbuf
.
- public void set_timeout (int timeout)
Sets the timeout of the notification.
- public void set_urgency (Urgency urgency)
Sets the urgency level of this notification.
- public bool show () throws Error
Tells the notification server to display the notification on the
screen.
- public bool update (string summary, string? body, string? icon)
Updates the notification text and icon.
Signals:
Inherited Members:
All known members inherited from class GLib.Object
- @get
- @new
- @ref
- @set
- add_toggle_ref
- add_weak_pointer
- bind_property
- connect
- constructed
- disconnect
- dispose
- dup_data
- dup_qdata
- force_floating
- freeze_notify
- get_class
- get_data
- get_property
- get_qdata
- get_type
- getv
- interface_find_property
- interface_install_property
- interface_list_properties
- is_floating
- new_valist
- new_with_properties
- newv
- notify
- notify_property
- ref_count
- ref_sink
- remove_toggle_ref
- remove_weak_pointer
- replace_data
- replace_qdata
- set_data
- set_data_full
- set_property
- set_qdata
- set_qdata_full
- set_valist
- setv
- steal_data
- steal_qdata
- thaw_notify
- unref
- watch_closure
- weak_ref
- weak_unref