MessageType
Description:
[ CCode ( cprefix = "GST_MESSAGE_" , type_id = "gst_message_type_get_type ()" ) ]
[ Flags ]
public enum MessageType
The different message types that are available.
Example: GStreamer concepts:
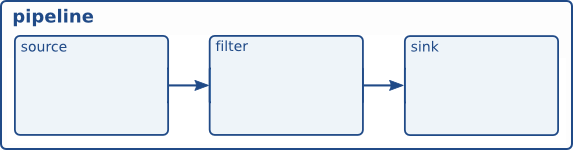
// See http://docs.gstreamer.com/x/ZgAF
// for a detailed description
public static int main (string[] args) {
// Initialize GStreamer:
Gst.init (ref args);
// Create the elements:
Gst.Element source = Gst.ElementFactory.make ("videotestsrc", "source");
Gst.Element sink = Gst.ElementFactory.make ("autovideosink", "sink");
// Create the empty pipeline:
Gst.Pipeline pipeline = new Gst.Pipeline ("test-pipeline");
if (source == null || sink == null || pipeline == null) {
stderr.puts ("Not all elements could be created.\n");
return -1;
}
// Build the pipeline:
pipeline.add_many (source, sink);
if (source.link (sink) != true) {
stderr.puts ("Elements could not be linked.\n");
return -1;
}
// Modify the source's properties:
source.set ("pattern", 0);
// Start playing:
Gst.StateChangeReturn ret = pipeline.set_state (Gst.State.PLAYING);
if (ret == Gst.StateChangeReturn.FAILURE) {
stderr.puts ("Unable to set the pipeline to the playing state.\n");
return -1;
}
// Wait until error or EOS:
Gst.Bus bus = pipeline.get_bus ();
Gst.Message msg = bus.timed_pop_filtered (Gst.CLOCK_TIME_NONE, Gst.MessageType.ERROR | Gst.MessageType.EOS);
// Parse message:
if (msg != null) {
switch (msg.type) {
case Gst.MessageType.ERROR:
GLib.Error err;
string debug_info;
msg.parse_error (out err, out debug_info);
stderr.printf ("Error received from element %s: %s\n", msg.src.name, err.message);
stderr.printf ("Debugging information: %s\n", (debug_info != null)? debug_info : "none");
break;
case Gst.MessageType.EOS:
print ("End-Of-Stream reached.\n");
break;
default:
// We should not reach here because we only asked for ERRORs and EOS:
assert_not_reached ();
}
}
// Free resources:
pipeline.set_state (Gst.State.NULL);
return 0;
}
valac --pkg gstreamer-1.0 concepts.vala
Content:
Enum values:
- ANY - mask for all of the above messages.
- APPLICATION - message posted by the
application, possibly via an application-specific element.
- ASYNC_DONE - Posted by elements when
they complete an ASYNC StateChange.
- ASYNC_START - Posted by elements when
they start an ASYNC StateChange.
- BUFFERING - the pipeline is buffering.
- CLOCK_LOST - The current clock as
selected by the pipeline became unusable.
- CLOCK_PROVIDE - an element notifies
its capability of providing a clock.
- DEVICE_ADDED - Message indicating a
Device was added to a
DeviceProvider (Since: 1.4)
- DEVICE_CHANGED - Message indicating
a Device was changed a
DeviceProvider (Since: 1.16)
- DEVICE_REMOVED - Message indicating
a Device was removed from a
DeviceProvider (Since: 1.4)
- DURATION_CHANGED - The duration of
a pipeline changed.
- ELEMENT - element-specific message, see the
specific element's documentation
- EOS - end-of-stream reached in a pipeline.
- ERROR - an error occurred.
- EXTENDED - Message is an extended message
type (see below).
- HAVE_CONTEXT - Message indicating that
an element created a context (Since: 1.2)
- INFO - an info message occurred
- INSTANT_RATE_REQUEST - Message
sent by elements to request the running time from the pipeline when an instant rate change should be applied (which may be in the past
when the answer arrives).
- LATENCY - Posted by elements when their
latency changes.
- NEED_CONTEXT - Message indicating that
an element wants a specific context (Since: 1.2)
- NEW_CLOCK - a new clock was selected in
the pipeline.
- PROGRESS - A progress message.
- PROPERTY_NOTIFY - Message
indicating a Object property has changed (Since: 1.10)
- QOS - A buffer was dropped or an element
changed its processing strategy for Quality of Service reasons.
- REDIRECT - Message indicating to request
the application to try to play the given URL(s).
- REQUEST_STATE - Posted by elements
when they want the pipeline to change state.
- RESET_TIME - Message to request
resetting the pipeline's running time from the pipeline.
- SEGMENT_DONE - pipeline completed
playback of a segment.
- SEGMENT_START - pipeline started
playback of a segment.
- STATE_CHANGED - a state change
happened
- STATE_DIRTY - an element changed state
in a streaming thread.
- STEP_DONE - a stepping operation
finished.
- STEP_START - A stepping operation was
started.
- STREAMS_SELECTED - Message
indicating the active selection of Streams has changed (Since: 1.10)
- STREAM_COLLECTION - Message
indicating a new StreamCollection is available (Since: 1.10)
- STREAM_START - Message indicating
start of a new stream.
- STREAM_STATUS - status about a
stream, emitted when it starts, stops, errors, etc.
- STRUCTURE_CHANGE - the structure
of the pipeline changed.
- TAG - a tag was found.
- TOC - A new table of contents (TOC) was found
or previously found TOC was updated.
- UNKNOWN - an undefined message
- WARNING - a warning occurred.
Methods: